Learn how to use a shared secret so we can validate the sensor data sent to a sensor endpoint.
If the data strategy selected for a sensor is "Endpoint", Nexudus waits for data to be sent to that endpoint. To validate this data actually originates from a trusted source, a shared secret is used to sign the request.
A share secret is automatically created for you when you register a new sensor. You can view and rotate this secret if needed within the details of each sensor
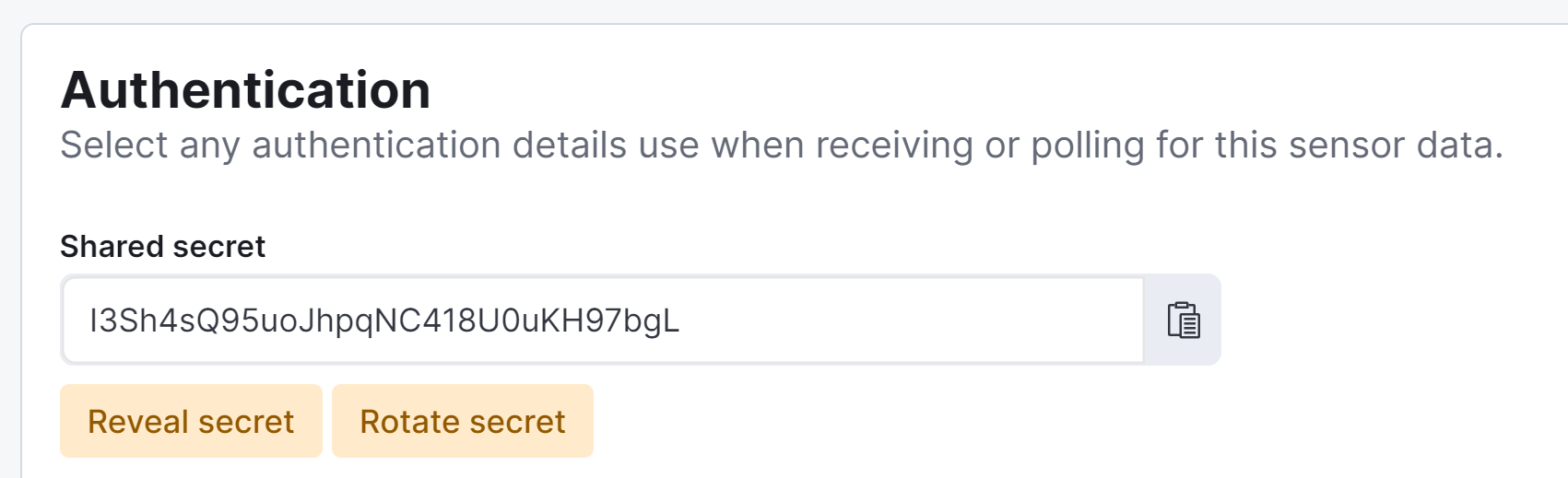
To validate the signature of a request, we serialise the payload of the request and calculate a HMACSHA256 signature using the shared secret. We will compare that signature with the value of the HTTP header X-Nexudus-Hook-Signature and only accept the request if there is an exact match.
Both the payload and shared secrets are converted to a byte array assuming UTF8 encoding. You may need to take this into consideration when computing the signature on your server in order to set the HTTP header.
var SIGNATURE_HEADER_NAME = "X-Nexudus-Hook-Signature"
var payload = Newtonsoft.Json.JsonConvert.SerializeObject(data);
if (payload == null) return Unauthorized();
var receivedHash = Request.Headers.GetValues(SIGNATURE_HEADER_NAME).FirstOrDefault();
if (receivedHash == null) return Unauthorized();
var keyByte = new UTF8Encoding().GetBytes(sharedSecret);
var messageBytes = new UTF8Encoding().GetBytes(payload);
var hmacsha256 = new HMACSHA256(keyByte);
var hashBytes = hmacsha256.ComputeHash(messageBytes);
var hash = ConvertByteToString(hashBytes);
if (hash != receivedHash)
return Unauthorized();